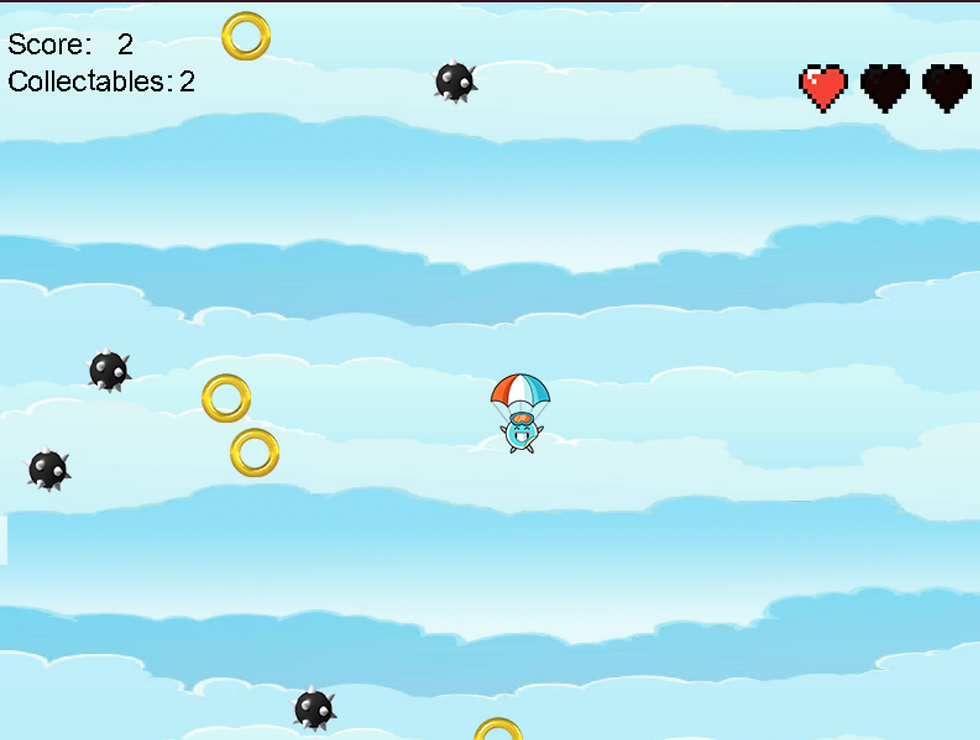
Skydiver
Skydiver is a C++ project written in SDL with focus on object-oriented programming and code architecture.
Content
1. Project Summary and Structure
2. Player Class and Input Processing
4. Generic Interactables Class and Child Classes
5. Game Manager
6. Conclusion
Project Summary and Structure
In Skydiver, the player moves vertically over the screen. The goal is to score the highest possible number by avoiding obstacles and collecting objects.
The project is written in C++ and utilizes SDL. The elements of it are, in general, textures rendered on rectangles.
The UML diagram below shows the dependencies and classes of the project:

Player Class and Input Processing
The player properties are defined in the player class. Here, the player is given a start position, and their position, should it be updated, is handled here. Furthermore, the player's health is initialized here.
Their input is processed in the input manager by means of the event handler.

Even though this could have also been put in the player class, it was separated to simplify the potential addition of a second player.
User Interface
Skydivers' user interface is managed in the respective class. To prevent memory leaks, the class contains a function that destroys the textures.
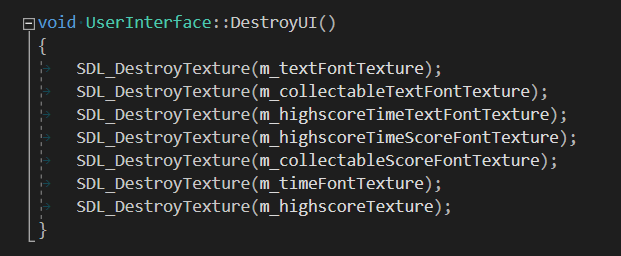
Most of the functions follow the same pattern, as a surface is created from which a texture is rendered, while the size of the rectangles is always dependent on the text. Meaning it is larger when there are more characters used. Every function that depicts text also frees the according surface and closes the font.

Generic Interactables Class and Child Classes
In Skydiver, the player can interact with two types of objects: Enemies and collectibles. These are child classes of a generic class that uses SDL's template class to minimize redundancy.
All objects are listed in an array that is initialized in the initialize function. This process depends on the size of the object itself and the player's position on the Y-Axis. Since the player can only move on the X-Axis, the object should not spawn over the player. To prevent similar spawn patterns of the objects, they are positioned using a seed that is generated upon loading the game.

The interactable objects move vertically across the screen, thus inevitably reaching a Y-axis position that is out of the visible range, as well as reaching an x value greater than the passed window width. If one of these circumstances arises, the interactable is again set to a random X value and the object therefore respawns.
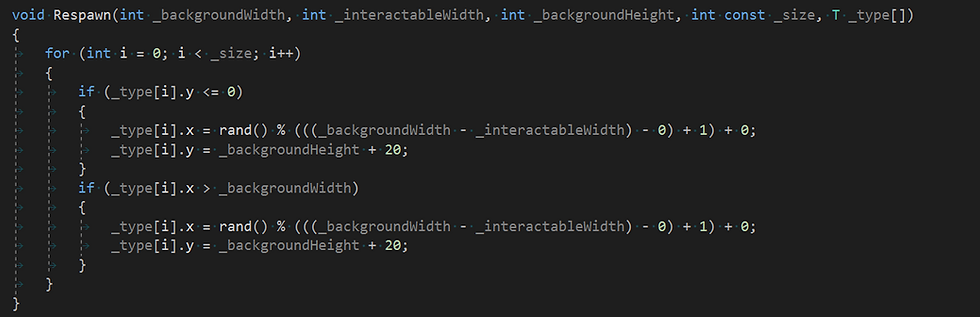
The vertical movement speed of the interactable is set depending on the delta time.

Two functions are implemented to detect a collision between two rectangles and act accordingly if the event occurs. The collision itself is calculated in the boolean function CollisionDetection.

If the return value is true, the object's x position is set to a value beyond the window. This triggers the respawn function.
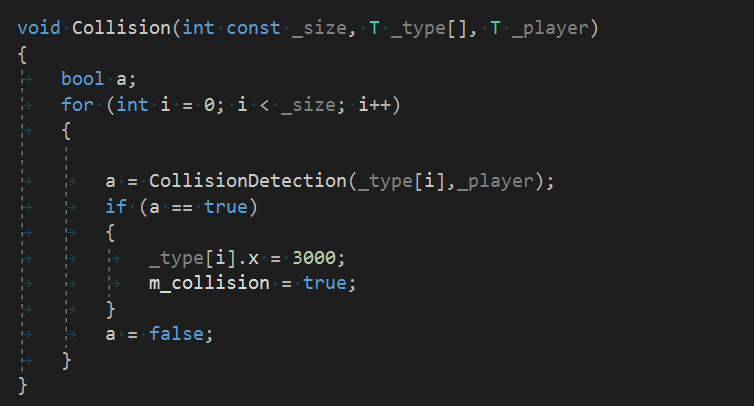
The enemies class is structured analogously to the collectibles class since both inherit from the same parent class. Both classes depict some redundancy, but in order to maintain the possibility of adding features, they were separated explicitly.
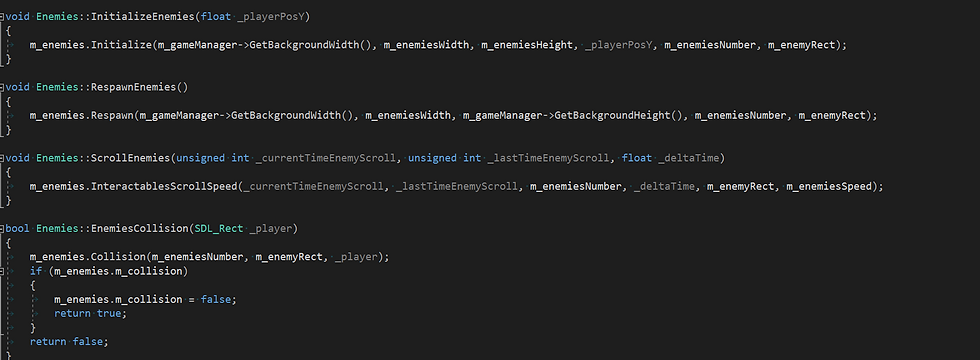
Game Manager
The game logic is managed by the game manager. The initializing of values, as well as the loading of images, the creation of textures, and the rendering, is managed here. The game loop is also defined here. So the movement of the player and the collision is called upon. Additional features such as the current score that is defined by the survived time are updated here.

The background is also updated by calling the background scroll function. To ensure endless scrolling, the background is rendered on two separate rectangles that are placed under each other should one reach the end of the screen.

As soon as the player's health reaches zero, the game over function is called.
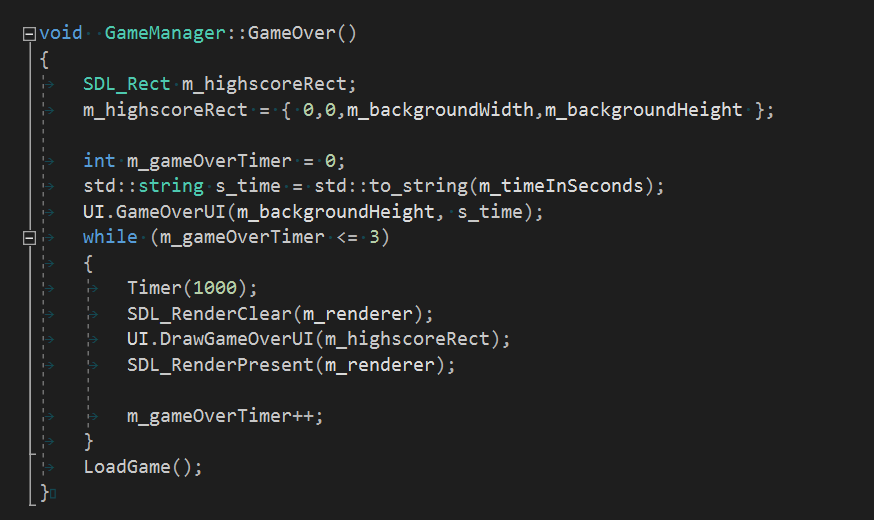
Conclusion
In conclusion, Skydiver is a small and working game that can be revisited and refined easily. Problems such as garbage collection and memory leaks were overcome, and preventive measures were taken, such as clearing surfaces and destroying textures at essential locations. Initially, the project was programmed to work and then refined in terms of, for example, the generic class. Features such as the scrolling background were added later to enhance the game's look and feel.
What went well
a learning success since it was my first C++ project
acquired knowledge in memory management and memory leak prevention
What went wrong
at first, problems with garbage collection and memory leaks
Project Gallery


